React Apollo Cannot Read .concat of Undefined
GraphQL is a new API standard that's a more effective, powerful, and agile substitute for the Residuum standard. GraphQL was developed equally an open-source projection in 2015 by Facebook. Today, the GraphQL language has a massive community of fans all over the world.
This language provides declarative information fetching, significant a client can specify exactly what data they need from an API. GraphQL is a query language for APIs, not a query linguistic communication for databases. A GraphQL server provides only i endpoint and responds with exactly the data the client has requested instead of providing several endpoints that render fixed data structures. Additionally, GraphQL simplifies the aggregation of data from different sources and uses specific types to describe queries and information.
In this article, we'll walk you through the main terms you need to know to piece of work with GraphQL and show you problems we've faced with GraphQL in our projects and ways to solve them.
GraphQL architecture
To understand the compages of GraphQL, we demand to know its main components.
Schema
A schema is the only thing that defines an API'due south capabilities and determines how clients tin can request information.
Resolvers
Resolvers are methods that render information for the current field.
Here's how a schema with a resolve method looks in GraphQL.
Types
A blazon is a way to describe information fields in GraphQL. This is what the primary types in the GraphQL architecture look like:
Output
Input
Enum
Interface
Unions
Custom scalars
Let's talk almost the GraphQL architecture and how it works. A client requests resource from a GraphQL server using a GraphQL query. Then the query is compared to the schema on the server according to its type. The GraphQL server analyzes the query, passes it recursively through the graph, and performs its resolver part for each field. When all requested data has been collected, the GraphQL server returns a response.
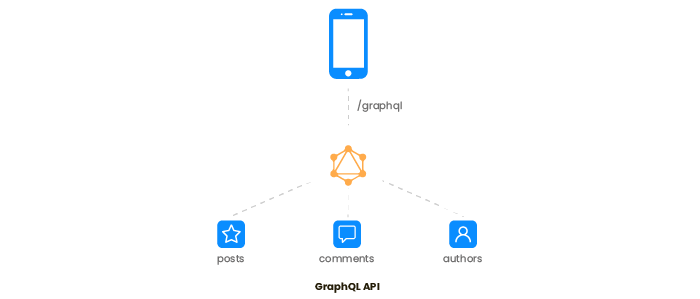
If you desire to use third-political party UI libraries to piece of work with a server, the procedure will be similar. First, yous'll generate a query using a tool like Apollo Client. And so GraphQL will start to aggregate information from entirely different sources (database, microservices, cache, etc.) based on this query. Here's a diagram of how information technology looks:
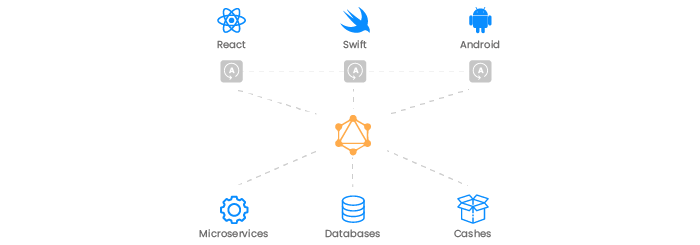
Apollo Customer
There are a lot of libraries you can utilise to work with GraphQL, including Relay, Prisma, URQL, and Apollo Client. Nosotros adopt to work with Apollo, as it has a large community of supporters, is often updated, and is compatible with iOS, Android, Angular, Ember, Vue, and React.
Apollo Client is a convenient client library for working with GraphQL. It can send queries, cache requested information, and update the state of UI components.
The Apollo Client library has a range of benefits:
- Declarative information option. You lot can write a asking and become data without needing to manually monitor downloads.
- Convenient instruments for development. You lot can take advantage of tools such every bit Chrome Apollo DevTools and VS Code plugins.
- Supports modern React and Hooks APIs.
- Universal compatibility. You can utilise any build settings and any GraphQL API.
- Strong community back up. This library is very pop and has a lot of committers who improve it all the time.
Now that we've checked out the architecture of GraphQL and chosen this library to work with, permit's review the types of queries in GraphQL.
Types of queries in GraphQL
GraphQL has some specific types of requests for unlike actions:
- Query — request to read data
- Mutation — request to change data
- Subscription — request that receives or sends existent-time effect data
Permit'south take a wait at each of these asking types in item.
1. Query
A query has a string structure that can incorporate parameters sent in the body of POST requests to the GraphQL endpoint. In response, we get a JSON file that contains either a information field with information nosotros requested or an "fault" field if something went wrong. For one request, we get i response.
Now let'south see how we can brand requests to Apollo Client.
1.1 Query with client object
A query with a client object is convenient as an boosted query in render mode. You can create a method in a component that will telephone call a query and return a response. In the example below, the argument of a client function is the property of a query component in Apollo Client.
A customer query can be executed as a side effect in a life cycle component. Apollo Client has a higher-lodge component (HOC) chosen withApollo, in which a client is the props of the wrapped component, as in the case below:
In this example, SOME_QUERY is a query cord to fetch data.
Now let's look at a request with a declarative description of query every bit a component.
1.two Query with a declarative description of query every bit a component
Equally you can see, the query in the lawmaking above returns iii properties: data, loading, and mistake. In our projection, we also used the refetch property, which allows us to refetch an existing query and get updated data. However, these aren't all the parameters the query can render. Y'all can read more nearly this in the useQuery API section of the Apollo Docs.
In the code snippet above, we showed a simple case of using queries. But what if nosotros have a more circuitous and interactive folio? Imagine we have a page that contains a circuitous course for editing and is divided into sections, where each modify is a commit to a database. As a outcome, you'll have nesting co-ordinate to the following construction.
This structure is extremely unreadable, peculiarly because of the layout and custom component. We demand to break this form into subcomponents. By doing so, we'll make the code more testable and readable. When taking a declarative approach, it'south better to apply a hierarchy with at most two layers of nesting. Tin we limit ourselves with the declarative arroyo to components? If you demand to apply a query in the lifecycle of React components, or if you need to pass requested data to a function that runs before the component is rendered, or if you take a composition of queries and mutations for one component, nosotros recommend using loftier-club components.
1.three Query with high-social club components
In the example beneath, we show the concept of using GraphQL with a single query.
And here'south an instance of using options, name methods, and config objects:
Hither, Company is a cord to fetch data. In that location are as well skip, props, withRef, and alias methods that tin exist described with a similar arroyo. You can read more about these methods in the Apollo Docs.
For united states, it's more interesting to accept a look at the composition of queries and mutations. To exercise this, we need to import the etch method. Here's how we do it:
Deport in mind that the etch method volition be removed in React Apollo Client 3.0. That's why it'southward better to use the flowRight method from the lodash library. Here'due south the proof.
Fetching information from the query looks like props of your components. Here's an case:
Hither'southward how information technology looks for class components:
Or if you're using functional components:
The list of returned parameters is like to declarative Query from subsection 1.two
1.four Queries with useQuery React Hooks
The new version of Apollo Client provides support for describing all query operations with the Hooks API. Hooks reduce the amount of code we demand to piece of work with data and brand components cleaner. As a result, our code is much easier to sympathise. Developers don't need to effigy out how high-order components or nested declarative components and their hierarchies are arranged. For greater consistency with hook components, nosotros recommend using Hook APIs if your project supports them.
The options contain all methods from the "query with the declarative description of Query every bit a component" subsection. If yous demand to add together parameters to the asking, it volition await similar this:
Here, NEW_EMAIL_REQUEST is a information query.
ane.5 Delayed useLazyQuery query
We're going to show you how to use a delay with the Claw API. Unlike useQuery, which is chosen when components are mounted, a request with a delayed phone call calls the request function but when an activity is performed. For instance:
Co-ordinate to this code, when all users are loaded, the getNewUser function is called, and a new user is requested when we click the button. We tin compare this with earlier versions of Apollo Customer:
In earlier versions of the Apollo Client library, we additionally needed to use the ApolloConsumer client object. As you can encounter from the lawmaking snippets, hooks simplify this implementation.
2. Mutation
A mutation is a asking to change data that has the structure of a cord. This string can exist with or without parameters. Information technology'southward sent in the body of POST requests to the GraphQL endpoint.
As a response, we go a JSON file that contains either requested data in the information field or an mistake field. For one request, we get one response.
2.1 Mutation request with client
In this case, the argument of the customer function is a Mutation property of the Apollo Client component. We can also access a customer object using the withApollo HOC or the mutation properties. Nosotros'll talk more about mutation options and properties in the next section.
two.2 Declarative description of a mutation
A declarative description is convenient and readable (in terms of the structure of the render props hierarchy) only at the starting time or maximum second level. This ways nosotros accept a major mutation that confirms the grade, and one chemical element of this class contains ane mutation. In all other cases, it's improve to use a mutation composition or Claw API. We'll get back to this later.
We also desire to testify y'all the options for refetchQuery, which updates the Apollo Client cache subsequently the mutation.
You can detect a total list of mutation options and backdrop in the Mutation section of the Apollo Docs.
2.3 Mutation with high-gild components
In the previous department, we saw that if we have more than 3 levels of nesting for declarative components, information technology'southward meliorate to utilize НОС to implement queries. Similar to Queries, Mutation requests likewise can be configured with an options object, like variables, name, and refetchQueries. Here'south an example of a mutation:
options.name
options.variables
options.refetchQueries
two.4 Asking with the useMutation hook
The useMutation claw in React is the main API for performing mutations in an Apollo Customer app. To get-go a mutation, we declare useMutation at the starting time of the React component and pass the GraphQL string (which represents the mutation) to the React component. When rendering a component, useMutation returns a function that we tin call at any time to perform the mutation along with an object with fields that represent the current state of mutation execution. For example:
In our projects, in the mutation options, we also use the onCompleted and onError callbacks to resolve successful and unsuccessful mutations.
three. Subscription
The 3rd type of operation bachelor in GraphQL is a subscription. There are cases when a client may want to get real-time updates from a server. A subscription allows us to catch GraphQL API events so we can modify data in real time. Data changes that clients can track are defined in the API every bit fields with a subscription type. Writing a GraphQL query to track a subscription is similar to defining other operations.
three.ane Description of a client subscription
In the example above, the argument of a customer part is a subscription property of the Apollo Client component.
3.two Declarative description of a subscription
Subscriptions are just listeners; they don't request whatever data when they're commencement continued, but they open a channel of connection for receiving information. Here's how we can visualize information:
For a total list of all subscription sections, options, and properties, bank check the Subscription department of the Apollo Docs.
3.3 Subscriptions with the useSubscription hook
Visualizing the subscription model with hooks is more than curtailed than declarative descriptions.
4. Request composition with the Hooks API
When nosotros execute multiple requests in one component, the use of high-lodge components or the render props mechanism tin can make our code difficult to understand. The fact that nosotros employ the render prop API and create structures with nested Mutation and Query components gives the deceptive feeling that our code is well-structured and has a clear hierarchy. Nosotros can avoid using НОС graphQL and additional methods like flowRight by declaring a Mutation at the starting time of components. The new useMutation and useQuery hooks allow u.s. to completely solve problems with a confusing hierarchy of render structures and eliminate the need to utilise HOCs
Here, we used additional methods such as flowRight, graphql hoc, and Query imported from dissimilar dependencies in package.json. The code gets more complex and less readable if the bodily contents of the return method are added. Nosotros can make the code cleaner and simpler with useQuery and useMutation.
We've gotten rid of unnecessary dependencies from other libraries, and the return method is clearer. The code is now far more structured and logical.
Carry in mind that in the official documentation for Apollo Customer 3.0, HOC and Components (<Mutation/> and <Query/>) volition have a notation that they're deprecated.
5. Queries with HTTP
Also using approved query types for receiving data, yous can get data with the aid of HTTP queries to GraphQL endpoints. Hither's how that looks:
We've described a query for fetching data in the query variable. The URL is the link by which the request volition exist executed. The variable opts contains options with which the request will be executed.
6. Mutation with HTTP
The variable mutate makes a request for getting a new accessToken. The variable URL is the link by which the request will be executed. The variable opts contains options with which the request will exist executed.
Customization
In this department, nosotros describe bug we've faced in our projects and demonstrate the solutions nosotros've come with when implementing different features.
Customization of HttpLink
In our projection, nosotros needed to customize an HttpLink object when implementing authorization and updating the user session token. The HttpLink object has a fetch method, which, by default, performs an HTTP asking to receive or alter information. We had to change the standard implementation of the fetch method. We can send mutations in the form of HTTP, every bit we mentioned, and thereby request or update an accessToken.
The implementation of our customFetch method looks similar this:
While implementing this method, it was impossible to use the customer object with a mutation because of a loop caused by the customFetch method of the HttpLink object.
Customization of FragmentMatcher
We needed to customize FragmentMatcher due to problems acquired by queries of the Union blazon. In the panel, we detected a "heuristic fragment matching going on!" warning.
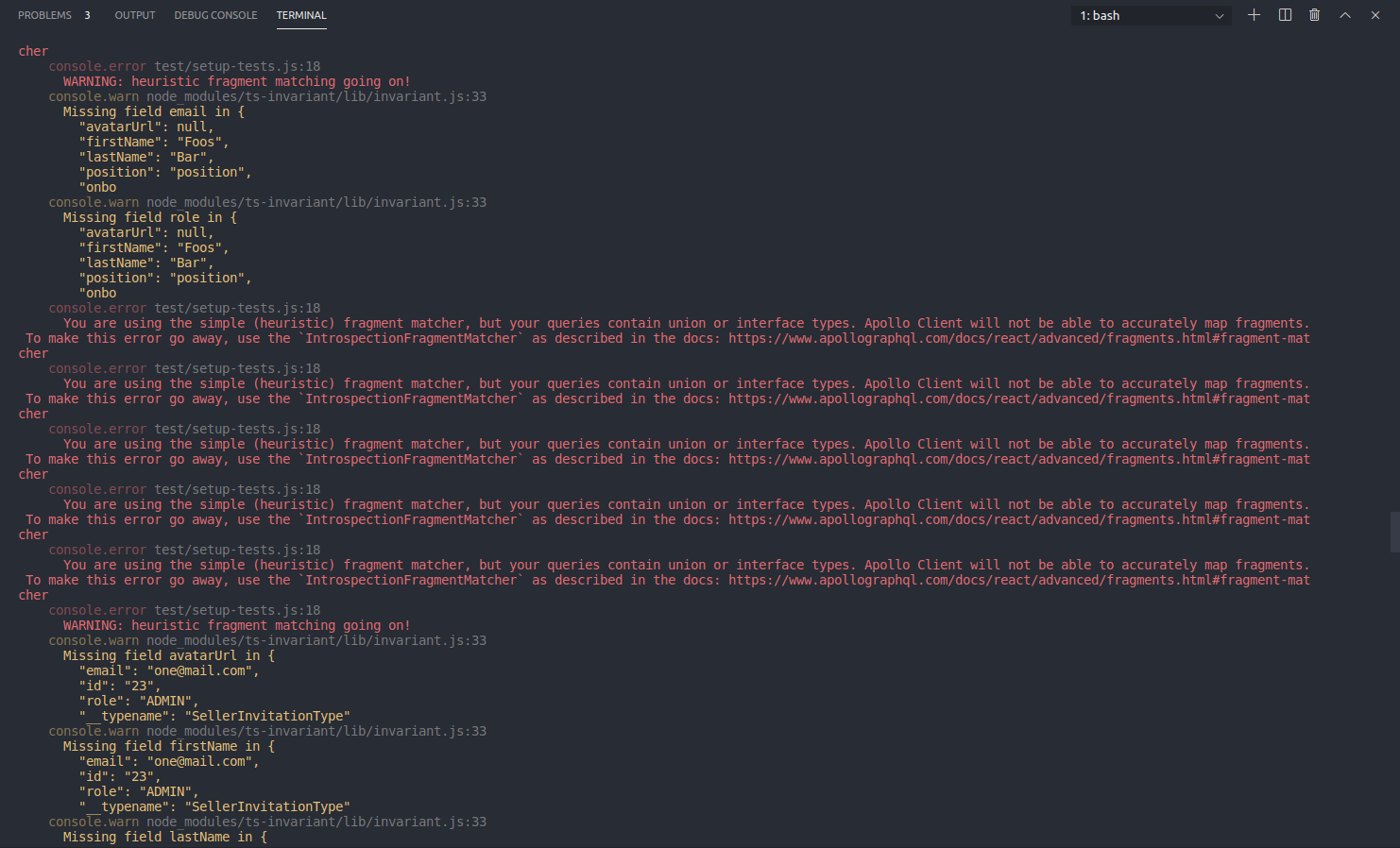
Before excavation into the cause of this problem, we need to realize that Apollo Client doesn't know our remote GraphQL schema and therefore tin't accurately display fragments. This means that in the console, we'll run into ane more error: "You are using the simple (heuristic) fragment matcher, but your queries comprise union or interface types."
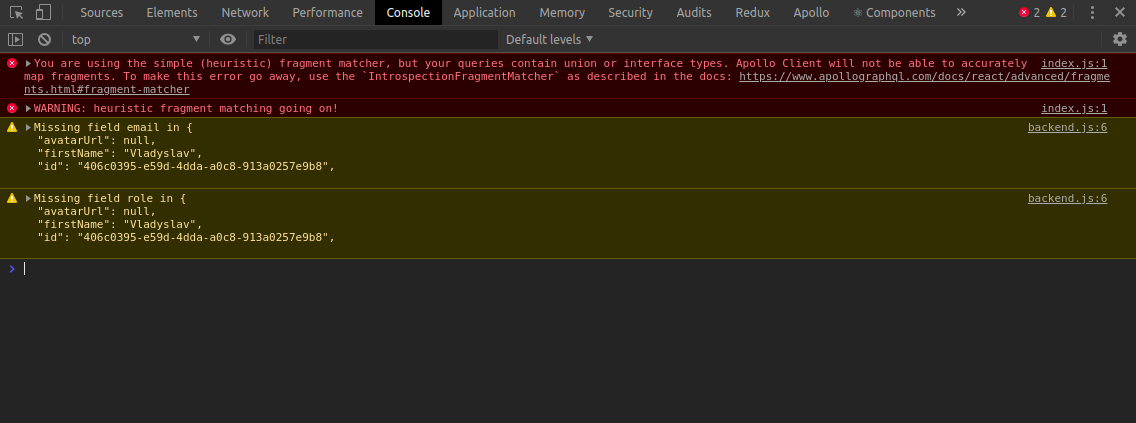
Now let's fix the cause of this warning.
Steps to get a remote GraphQL schema locally
#i Request a schema
We need to request a GraphQL schema from the back cease and save information technology locally.
We saved the file as src/scheme/fetchScheme.js. We demand this file because in packet.json, we will execute the control to update the schema in society to maintain its relevance.
#ii Laissez passer localGqlScheme to InMemoryCache
At present we need to laissez passer the fragmentMatcher object to the ApolloClient constructor.
#3. Build and request the scheme in localGqlScheme.json
We apply yarn and Side by side.js in our project, request a schema, and build the project.
#iv. Testing after implementing fragmentMatcher
In the previous section, we successfully implemented fragmentMatcher. Notwithstanding, all our tests will fail because the testing environment doesn't know the schema we put in the cache.
When you make all the changes shown above, your tests will be successful because MockedProvider will work with the relevant cache. Think that in every case when you demand to exam cases with mock queries of the Union type, you need to add __typename.
In all other cases without Wedlock or Interface, the type name is optional. In the example above, you can see that the withTypeName properties tin be configured.
Final thoughts
In this article, we explained what GraphQL is, demonstrated the popularity of Apollo Client for working with GraphQL, showed how Apollo Client interacts with the UI and GraphQL, and, what'southward most important, demonstrated diverse methods for describing requests and subscriptions in Apollo React Client. We shared with you not merely numerous means to describe types of operations just as well our own ideas and experience gained in our projects.
If you lot take any comments or suggestions, commencement a conversation below.
Source: https://rubygarage.org/blog/graphql-in-react-with-apollo-client
0 Response to "React Apollo Cannot Read .concat of Undefined"
Post a Comment